Interested in learning Python? Jack Wallen takes you through your first steps in building a simple application to take user input and write it to a file.
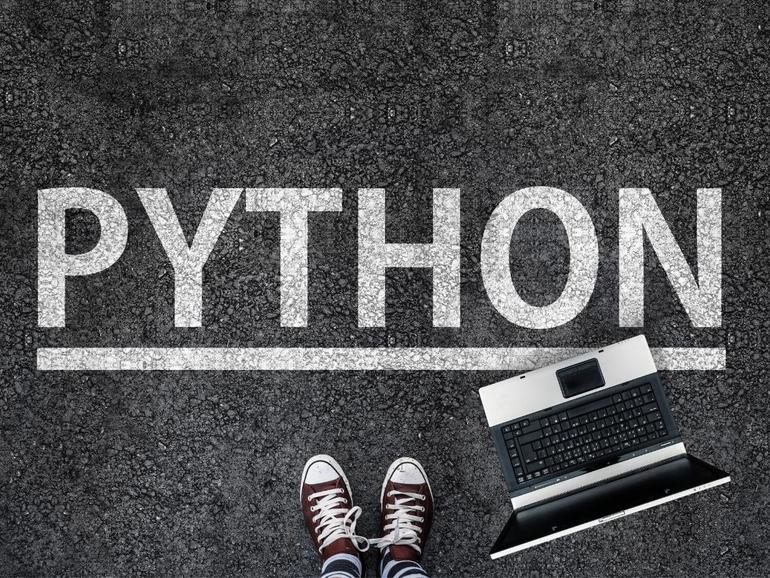
Image: iStockphoto/mirsad sarajlic
Python is an open source, interpreted, high-level programming language used for general-purpose development. Unlike many languages, Python doesn’t require a compiler, which makes it an incredibly efficient language to work with. You can write code and run it directly from the command line.
But, Python’s efficiency doesn’t just come from its lack of a compiler dependency. Python makes the debugging of code very easy. When you run your Python app, if the interpreter runs into an error in the code, it will present a very clear indication as to what is wrong, on a line-by-line basis. Because of that, Python is one of the easier languages to learn. It’s also why so many, new to programming, opt to start with Python.
I want to give you an easy-to-understand introduction, by way of creating an application that will take input from the user and store that input in a file. What we’ll create is a simple command-line application to store software licences.
I’ll be demonstrating on Pop!_OS Linux, but this will work on any platform that supports Python.
SEE: Python is eating the world: How one developer’s side project became the hottest programming language on the planet (cover story PDF) (TechRepublic)
What you’ll need
In order to make this work, you’ll need an operating system that supports Python 3. I’ll show you how to install Python 3 on Linux. If you use a different operating system, you’ll need to modify the installation instructions to suit your platform of choice.
SEE: Hiring kit: Python developer (TechRepublic Premium)
How to install Python 3
As of January 1, 2020, Python 2 was officially sunsetted. Although you can install Python 2, you’ll find that some of its features are not backwards compatible with Python 3. Because of that, you must install Python 3.
The first thing you must do is install the latest version of Python. To do that, open a terminal window on your Linux machine and issue the command:
sudo apt-get install python3 -y
If you’re using a Red Hat-based distribution, that command would be:
sudo dnf install python3 -y
If you’re working with either macOS or Windows, download the required installer for your platform from the official Python download page.
To verify the installation succeeded, issue the command:
python3 --version
You should see the version number of Python printed out (Figure A).
Figure A
” data-credit rel=”noopener noreferrer nofollow”>
Python 3.8.2 is installed on Pop!_OS Linux.
How to create your first Python app
Let’s cut to the chase and create our first app. As I mentioned, we’re going to create an app that takes user input in the form of Software Title and Software License. That input will then be written to a file named licenses.txt.
The first thing we’ll do is create a new directory to house our Python projects. Issue the command:
mkdir ~/PYTHON
Change into that new directory with the command:
cd ~/PYTHON
Our app code will be broken into three sections:
-
Input: Define the functions for user input
-
File: Define the file to be used to save the output
-
Output: Define how our output is to be formatted
In order to take input from the user, we’ll define two variables:
- input1: Used for the Software Title input
- input2: Used for the Software License input
Our first two lines of code are simple variable declarations for input1 and input2:
input1 = input("Software Title:") input2 = input("License Number:")
Believe it or not, that’s our first section of code. When the program asks for input1 data, it will clearly print out what the data is for (Software Title). The same holds true for input2 (Software License). Of course, we’ll add comments to make it easy for anyone to understand.
The next section defines the file that will save our user input. A normal Python 3 statement to define an output file looks like:
file = open("filename")
However, we need our file to append new input to the end of the file, otherwise, every time the app is used, it’ll overwrite the previous input. For that, we use the “a” option for append. So now our file open section looks like:
file = open("licenses.txt", "a")
Next comes the final (and largest) section of our app, the output. What we’re going to do is define the output such that it is in the form of:
Software Title:Software License ---
Every entry will be separated by three dashes for clarity.
What does this section look like? We’ll be using our variables (input1 and input2) from the input section, in conjunction with the file.write function (which writes input to a file). The first line is:
file.write(input1)
This will take whatever the user types for input1 and save it to the licenses.txt file.
The next line is:
file.write(":")
The above line writes a colon directly after the input1 variable. Anything in quotes is actually printed directly to the output file.
Next, we take the input from the input2 variable and write it directly after the colon. In addition to that, we’re going to add a new line option (to add a carriage return), so after the final input, the next file.write will start on a new line. This is done with:
file.write(input2 + "\n")
The “\n” is read as a new line in Python3.
Our next line writes out the dashes and adds a new line like so:
file.write("---" + "\n")
Lastly, we close out the file write session with the line:
file.close()
And that’s it for our Python3 code. We add comments so that the entire file looks like:
# THIS PROGRAM READS LICENSE INPUT FROM USER # AND OUPUTS IT TO THE FILE license.txt # FIRST WE TAKE INPUT FOR THE SOFTWARE TITLE input1 = input("Software Title:") # NEXT WE TAKE INPUT FOR THE SOFTWARE LICENSE input2 = input("License Number:") # NOW WE DEFINE THE FILE TO SAVE OUTPUT INTO # WE USE THE "a" OPTION TO APPEND TO THE FILE # OTHERWISE EACH INPUT WOULD OVERWRITE THE # PREVIOUS INPUT file = open("licenses.txt", "a") # WE USE A NUMBER OF file.write STATEMENTS TO WRITE THE OUTPUT IN A FORMAT # SUCH THAT THE SOFTWARE TITLE AND LICENSE IS SEPARATED BY A : # AND EACH TITLE/LICENSE IS SEPARATED BY A --- file.write(input1) file.write(":") file.write(input2 + "\n") file.write("---" + "\n") file.close()
All Python apps end with the .py extension, so create a new file with the command:
nano license.py
Copy and paste the entire code into the newly-created license.py file. Save and close the file.
How to run a Python app
Now we can run our app, using the python3 command like so:
python3 license.py
You will first be asked for the Software Title, followed by the Software License (Figure B). After entering the Software License the app will close.
Figure B
” data-credit rel=”noopener noreferrer nofollow”>
The interaction of our Python application.
You can then view the licenses.txt file to see that the new input has been appended to the end of the file (Figure C).
Figure C
” data-credit rel=”noopener noreferrer nofollow”>
Sample input for our license.py Python application.
And that’s it. A very simple Python application to takes user input and writes it to a file. As you can see, Python is a very easy programming language to get started with. And using this simple example, you can expand on it and begin to build your own useful Python programs.
Also see
Source of Article