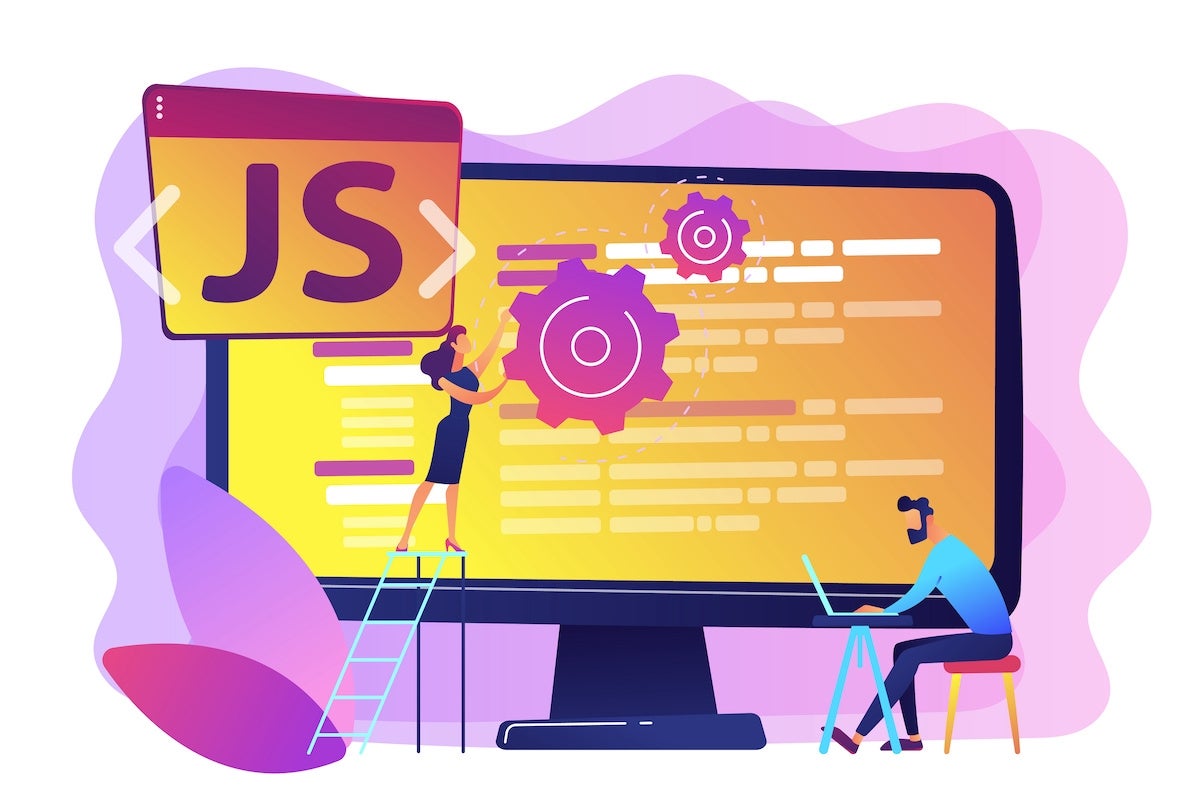
JavaScript is a versatile and widely used programming language that powers dynamic web applications. As development projects become more complex, following best practices becomes essential to ensuring maintainability, scalability and code quality. In this article, we’ll explore a comprehensive set of best practices for JavaScript development, accompanied by code examples.
Jump to:
1. Consistent code formatting
Consistency in code formatting enhances readability and collaboration. Adopt a consistent coding style and use tools like ESLint and Prettier to automate formatting.
// Inconsistent formatting
function exampleFunction(){
const result=10*2;return result; }
// Consistent formatting
function exampleFunction() {
const result = 10 * 2;
return result;
}
2. Use strict mode
Enable strict mode to catch common coding mistakes and promote better practices by preventing the use of undeclared variables, assigning to non-writable global variables and more.
‘use strict’;
// Omitting the var keyword is OK in non-strict mode
x = 10;
// In strict mode the above variable declaration throws an error:
// Uncaught ReferenceError: x is not defined
3. Variable declarations and scoping
Forget the outdated var keyword; use let and const for variable declarations to control scoping and prevent unintended reassignment. Employ const for variables that won’t be reassigned.
// Good
let counter = 0;
const maxAttempts = 5;
// Avoid
var totalCount = 0;
4. Avoid global variables
Minimize the use of global variables to prevent polluting the global scope. Encapsulate your code in functions or modules to limit variable exposure.
// Avoid
let globalVar = ‘I am global’;
function someFunction() {
globalVar = ‘Modified’;
}
SEE: Top Online CSS and HTML Courses
5. Function declarations and expressions
Use function declarations for named functions and function expressions for anonymous functions. Function declarations are hoisted, while function expressions are not. Hoisting is the default behavior of moving all the declarations to the top of the scope before code execution.
// Function Declaration
function add(a, b) {
return a + b;
}
// Function Expression
const multiply = function(a, b) {
return a * b;
};
6. Naming conventions
Choose meaningful and descriptive names for variables, functions and classes. Follow conventions like camelCase for variables and functions and PascalCase for classes.
const userName = ‘JohnDoe’;
function calculateTotalPrice() {
// …
}
class UserProfile {
// …
}
7. Commenting and documentation
Write clear comments to explain complex logic, assumptions or potential pitfalls. Document your code using tools like JSDoc to generate formal documentation.
// Calculate the area of a rectangle
function calculateRectangleArea(width, height) {
return width * height;
}
/**
* Represents a user profile.
* @class
*/
class UserProfile {
// …
}
8. Error handling
Implement proper error handling to make your code more robust and prevent crashes. Use try-catch blocks for synchronous code and .catch() for promises.
try {
// Risky code
} catch (error) {
// Handle the error
}
somePromise
.then(result => {
// …
})
.catch(error => {
// Handle the error
});
SEE: jsFiddle: an online playground for your JavaScript, CSS, and HTML
9. Modularization and dependency management
Break down your code into smaller, reusable modules. Use tools like ES6 modules or CommonJS for better organization and maintainability.
// math.js
export function add(a, b) {
return a + b;
}
// app.js
import { add } from ‘./math.js’;
console.log(add(2, 3)); // Output: 5
10. Asynchronous programming
Utilize callbacks, promises or async/await to manage asynchronous operations. This ensures smoother execution and better error handling.
// Using Promises
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => {
// Handle data
})
.catch(error => {
// Handle error
});
// Using async/await
async function fetchData() {
try {
const response = await fetch(‘https://api.example.com/data’);
const data = await response.json();
// Handle data
} catch (error) {
// Handle error
}
}
11. Optimizing performance
Optimize your code for better performance by reducing unnecessary computations, minimizing DOM manipulations and optimizing loops.
// Inefficient loop
for (let i = 0; i < array.length; i++) {
// …
}
// Efficient loop
const length = array.length;
for (let i = 0; i < length; i++) {
// …
}
12. Testing and quality assurance
Write unit tests using frameworks like Jasmine and Jest to ensure your code behaves as expected and catch regressions early. Utilize linters and static analysis tools to identify potential issues.
// Using Jest for testing
function add(a, b) {
return a + b;
}
test(‘add function adds two numbers’, () => {
expect(add(2, 3)).toBe(5);
});
Final thoughts on best practices for JavaScript development
Adopting these best practices can significantly improve the quality, maintainability and performance of your JavaScript code. By focusing on consistent formatting, scoping, error handling and other key aspects, you’ll be well-equipped to tackle complex projects with confidence. Remember that best practices may evolve over time, so staying up-to-date with the latest trends and tools is also crucial for continued success in development.
Source of Article